Selenium
First we want to ensure that we have Rollbar instrumented in the application (View Rollbar SDK’s), if you already have Rollbar instrumented you are moments away from a debugging life-changing experience.
Next, we want to notify Rollbar when a selenium test is executing so we can link the test failure (when it occurs) to the Rollbar errors detected at the time of the test execution.
Here is a basic example of injecting the Selenium SessionId into the Rollbar SDK during the test execution. In this example we will fetch the selenium sessionid and inject it into the web application using the ExecuteScript function.
The sessionid comes from the selenium remote web driver object.
The important line of code is this one:
_driver.ExecuteScript("Rollbar.configure({ payload: { 'seleniumsessionid' : '" + _driver.SessionId + "' } })");
Example Test:
[Test]
public void InvalidLoginAuth()
{
//Use driver to open Page
_driver.Navigate().GoToUrl("https://test.app.com/login");
_driver.ExecuteScript("Rollbar.configure({ payload: { 'seleniumsessionid' : '" + _driver.SessionId + "' } })");
IWebElement emailInput = _driver.FindElement(By.Id("email"));
IWebElement passwordInput = _driver.FindElement(By.Id("password"));
IWebElement loginButton = _driver.FindElement(By.Id("btnLoginAuth"));
emailInput.SendKeys("[email protected]");
passwordInput.SendKeys("test1234");
loginButton.Click();
var lastRollbaruuid = _driver.ExecuteScript("return _lastRollbaruuid;");
_driver.ExecuteScript("browserstack_executor: {\"action\": \"setSessionStatus\", \"arguments\": {\"status\":\"failed\", \"reason\": \" View Error in Rollbar https://rollbar.com/occurrence/uuid/?uuid=" + lastRollbaruuid + " \"}}");
Assert.Fail();
}
That is it! Your test executions can now continue normally without any modifications to your selenium scripts.
Service Links To Test Automation Providers:
We now have a much clearer understanding of the exact reason that this specific test failed without having to re-run the test, or try to debug it locally.
Rollbar can also link back to the test execution report in most popular cloud vendors like Browserstack, Perfecto, SauceLabs, Digital.ai and others using Service links from within Rollbar.
Service Links Examples:
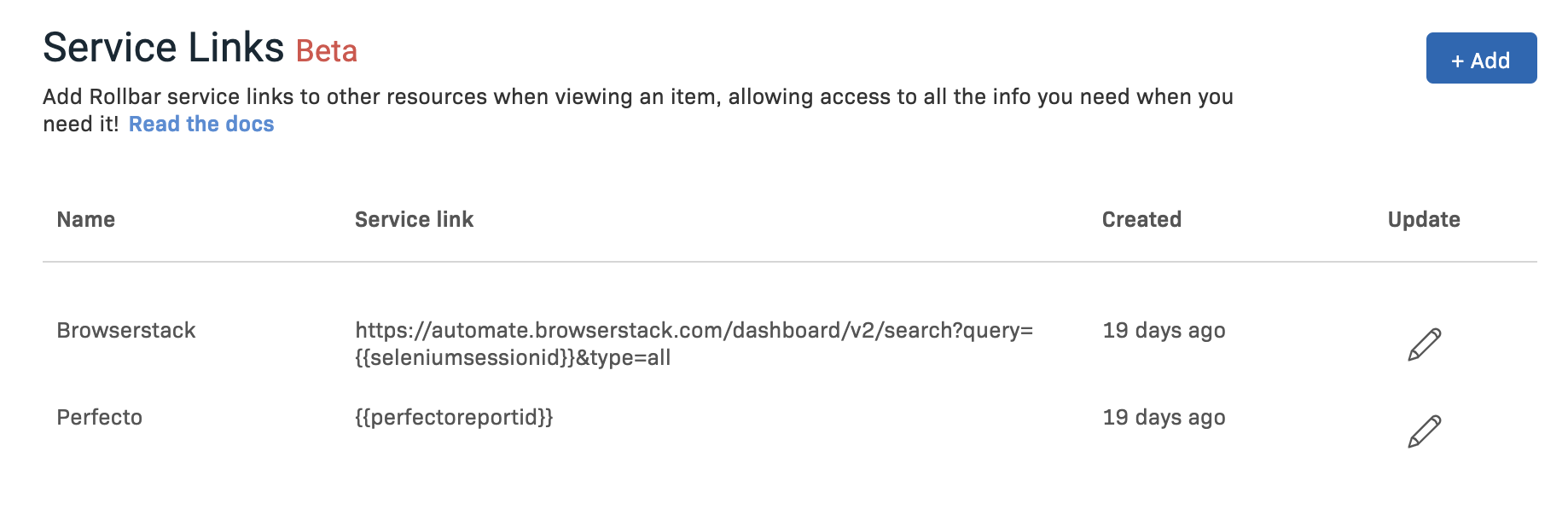
Use RQL To Find all errors with Selenium SessionId
Using RQL you can now easily find all errors that occurred during a specific selenium session using this query.
SELECT *
FROM item_occurrence
WHERE seleniumsessionid='XXXXXXX'
Updated 12 months ago