LaunchDarkly
Introduction
Enabling the LaunchDarkly integration allows engineers to automate Feature Flag toggles based on errors captured in Rollbar. This means that if you ship a feature to users, only 1 user will see an error before Rollbar automatically toggles the feature flag for all subsequent users.
Example
When you're working on code behind a Feature Flag, you will add an additional key-value pair specifying this Feature Flag with the standard error payload you send to Rollbar. If you have the LaunchDarkly integration enabled, and we receive an item that matches a rule you specified within the integration. (e.g. environment: production), we will take an action defined within the rule (e.g. turn OFF the specified feature flag).
Setup Instructions
On LaunchDarkly
- Get an API access token by navigating to the LaunchDarkly website, Account Settings > Authorization, and click +Token.
- When creating the token, set Role to "Writer", because this token will update feature flag settings.
- Copy the access token, because it will be hidden in the future within LaunchDarkly.
- Finally, you can create a new feature flag, or take note of an existing one you plan on using.
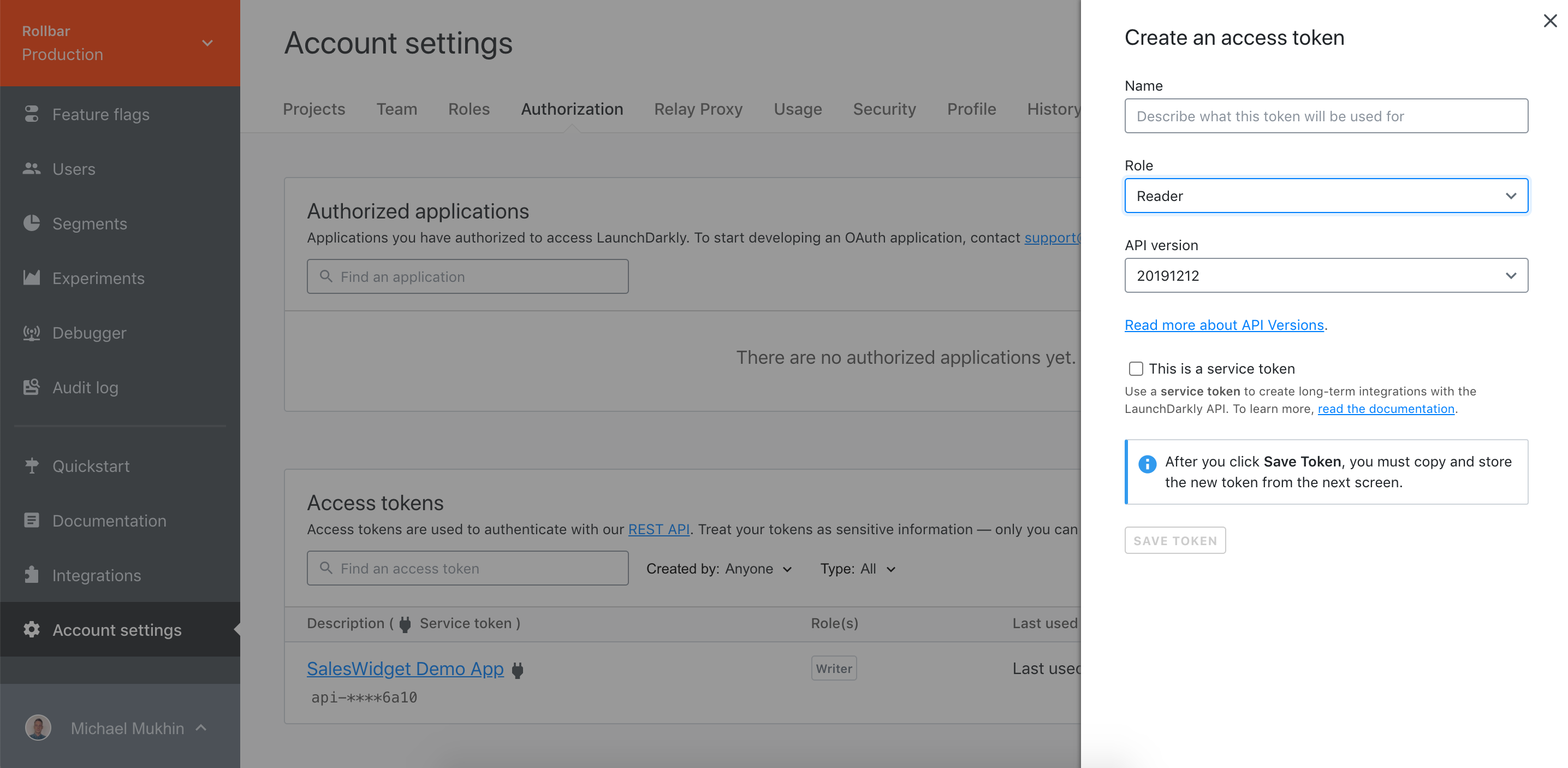
In Rollbar Settings
- Choose a Project and navigate to Project Settings > Notifications
- Click on the Launch Darkly integration
- Add the Launch Darkly API token, and Save
- Create a rule
- Select the project, environment and feature flag you have created in Launch Darkly
- Select the action to be taken with the feature flag (e.g. turn it off)
- Add filters
- If these filters are matched for a new item, the action above will be triggered for your chosen Feature Flag
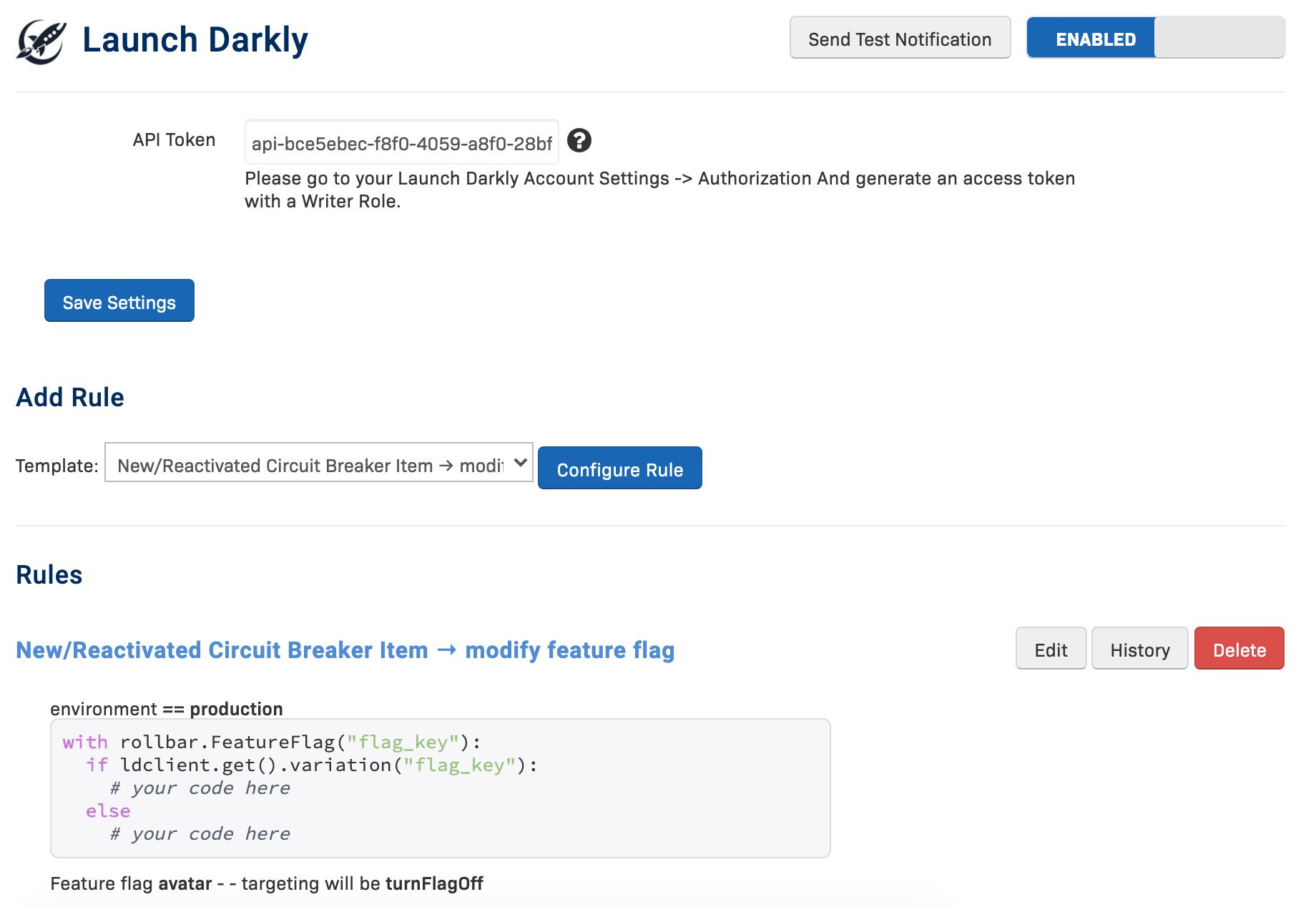
In Your Code
You must send an additional key-value pair that identifies the Feature Flag, along with the standard error payload.
If you are using Python, please update the SDK here. For other languages, we have provided examples using a Transform function.
# For Python, you can call our new context manager interface in the Python SDK
# to conveniently tag any payload being sent to Rollbar with feature flag data.
with rollbar.feature_flag('YOUR_FEATURE_FLAG_KEY'):
do_some_risky_code()
# For Ruby, you can set a new scope with the tags object, so that any errors
# or logs reported within that scope will also include the tags object.
scope = {:tags => [
{:key => "feature_flag.key", :value => "YOUR_FEATURE_FLAG_KEY"}
]}
Rollbar.scoped(scope) do
begin
do_some_risky_code()
rescue => e
# Sends a report with the above feature flag data under the tags key
Rollbar.error(e)
end
end
/**
* For PHP setups, you'll want to write a custom transformer and a custom data
* object. The custom transformer will utilize the custom data object by taking the
* feature flag key passed in the extra data and format it and place it under the
* tags key in the custom data. This custom data will take care of serializing the
* payload before the custom transformer returns it to be sent to Rollbar.
*
* For a more in depth code example, check it out the examples repo:
*
* https://github.com/rollbar/rollbar-php-examples/tree/master/tags-transformer
*
*/
// For Browser-js setups, you could pass the information as extra data and
// then use the transform option in configuration to move it to the root level
// of the payload like so:
try {
doSomeRiskyCode();
} catch (e) {
// Passing the `feature_flag_key` as extra data
Rollbar.error(e, {feature_flag_key: 'YOUR_FEATURE_FLAG_KEY'});
}
...
// The config:
var _rollbarConfig = {
// The usual
transform: function(payload) {
body = payload.body.trace || payload.body.message;
// `feature_flag_key` must match the extra data being sent in the code above.
if (body.extra && body.extra.feature_flag_key) {
payload.tags = [{
key: 'feature_flag.key',
value: body.extra.feature_flag_key
}];
delete body.extra.feature_flag_key;
}
}
}
// For Node.js, extra data passed with an explicit call to Rollbar should be
// placed at the root level of the payload, so the code below should suffice.
try {
doSomeRiskyCode()
catch (e) {
rollbar.error(e, {'tags': [
{'key': 'feature_flag.key', 'value': 'YOUR_FEATURE_FLAG_KEY'}
]});
}
// For any other SDK, as long as there is a transform option in the configuration,
// you can still get this set up. All you would need to do is (pseudo code below):
// 1. send the extra data along with the error payload
Rollbar.error(
'Oh no! An error occurred',
{'feature_flag_key': 'YOUR_FEATURE_FLAG_KEY'} // this is the extra data
)
// 2a. write a transform method to grab the extra data and set it to under the 'tags'
// key at the root payload
def transform(payload):
if payload['data']['custom']['feature_flag_key']:
value = payload['data']['custom']['feature_flag_key']
payload['tags'] = [{'feature_flag.key': value}]
// 2b. and in your configuration, specify the transform!
Rollbar.init(
YOUR_ACCESS_TOKEN,
transformer: transform
)
To test, send an item to Rollbar, and in the UI check if the occurrence has the key tags
in the payload. tags
should be a list of objects where each object contains a key
and a value
. The key
should match 'feature_flag.key'
and the value
should match your feature flag key. If you see the corresponding tag as the last element in tags
, a Rule for the Launch Darkly integration will be triggered if the filters match the occurrence you sent.
Notifications
When this integration successfully executes, we add a comment to the Launch Darkly feature flag change that contains a link to the Rollbar Occurrence which triggered the action. We find it very useful to set up a Launch Darkly + Slack integration which notifies us whenever a flag is changed - we suggest you consider this.
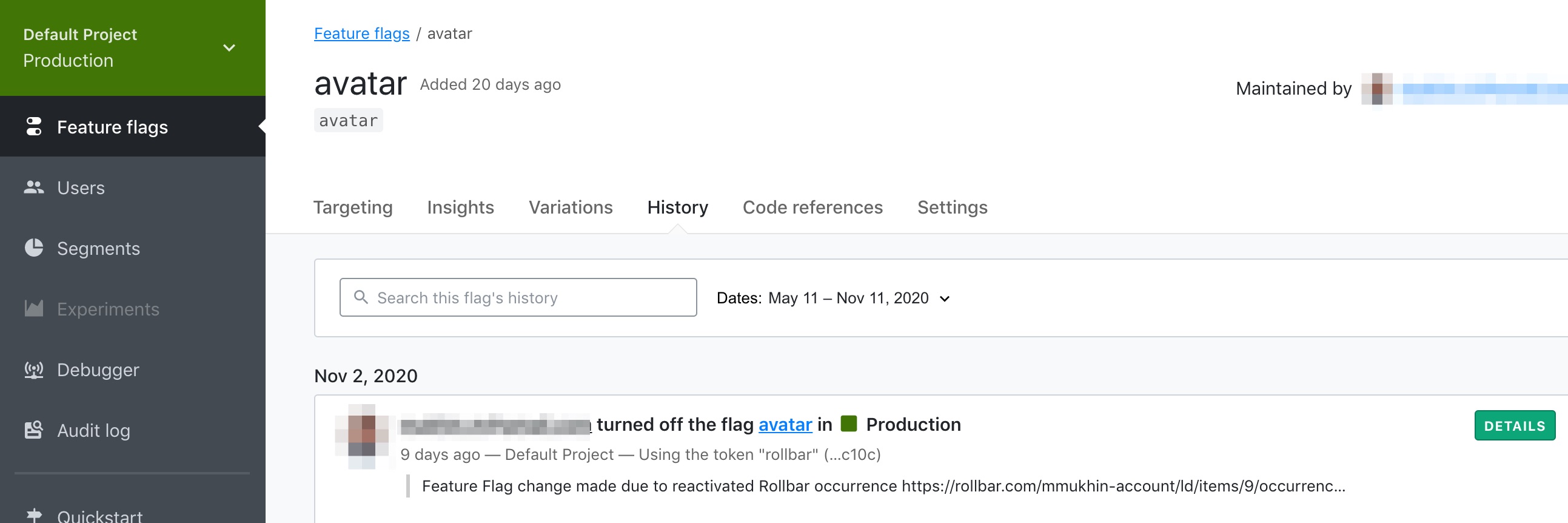
Troubleshooting
- The feature flag name you send via the SDK must match exactly to the feature flag you select in the integration
Updated 5 months ago